Negative Testing In Software Testing | How To Perform Negative Testing
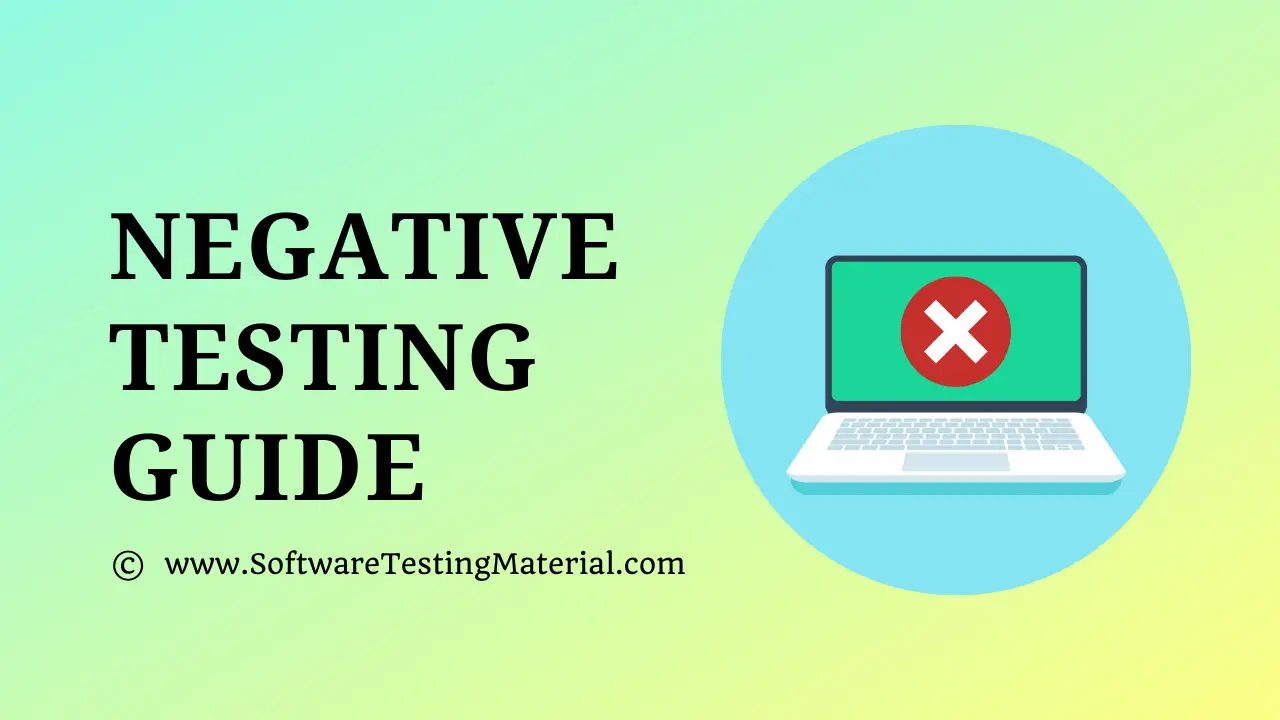
In this article, we will see what is negative testing with some example negative test scenarios. The article contains the following sections:
Two of the main testing strategies in software testing are negative testing and positive testing.
What is Negative Testing in Software Testing with Examples?
Negative testing is also known as Failure testing or Error path testing is a technique used in software testing to ensure that the application can gracefully handle invalid input or unexpected user behavior. The primary goal is to determine the robustness and stability of the software by inputting invalid data, incorrect user actions, or unexpected conditions. Essentially, negative testing checks if the software breaks or behaves inappropriately when faced with negative scenarios.
Examples of Negative Testing (Negative Testing Scenarios):
Negative testing helps ensure that the software can handle unexpected or invalid inputs. Here are some negative scenarios in testing with examples:
Example #1. Phone Number Field Negative Test Scenario
Suppose you are testing a form that requires a phone number. Instead of entering a valid phone number like “123-456-7890”, you enter a string of letters such as “abcdefg”. The system should properly handle this invalid input by displaying an error message.
- Scenario: Entering special characters or alphabetic characters in the phone number field.
- Example: Inputting “@!#123abcd” into the phone number field.
- Scenario: Inserting fewer or more characters than required.
- Example: Inputting “12345” (less than 10 characters) or “123456789012” (more than 10 characters).
Example #2. Age Field Negative Test Scenario
The test case for negative testing could be to input age as the alphabet or negative integer.
- Scenario: Inputting negative numbers or non-numeric values into the age field.
- Example: Entering “-25” or “twenty-five”.
- Scenario: Providing values beyond logical age limits.
- Example: Inputting “150” in the age field.
Example #3. Zip Code Field Negative Test Scenario
Zipcode format varies in different countries. Negative test cases in such scenarios could be input alphanumeric value for the USA, India, and numeric for Canada, UK. Exceeding the number of characters in the zip code field is also a negative test case.
- Scenario: Entering alphabetic characters or special characters in the zip code field.
- Example: Inputting “ABC123” or “#@!567” into the zip code field.
- Scenario: Providing a zip code that is significantly longer or shorter than the standard format.
- Example: Inputting “1234” (less than 5 digits) or “123456789” (more than 5 digits).
Example #4. Mandatory Field Negative Test Scenario
Test by skipping the required data entry and try to proceed further.
- Scenario: Leaving a mandatory field blank or entering irrelevant data.
- Example: Submitting a form without entering any data in a mandatory ‘Name’ field, or entering numbers like “12345” in the name field.
Example #5. For Data Bounds and Limits Negative Test Scenario
Enter large values to test the size of the fields.
- Scenario: Providing excessively large values that exceed the maximum limit.
- Example: Inputting 1,000,000 characters into a text box meant to accept up to 255 characters.
Other Examples
- Testing Boundary Values: If a field demands a user to input a password between 8 and 16 characters, and you enter a password with 7 or 17 characters, the application should reject these inputs and alert the user that the password length is invalid.
- Testing Unexpected User Actions: In an online shopping application, selecting an item and attempting to delete it from the shopping cart while the network is disconnected. The system should communicate the error clearly and guide the user on the next steps, rather than crashing or freezing.
- Testing File Uploads: Trying to upload an unsupported file type, such as a .exe file, in a field that only accepts .jpg or .png images. The software should block this action and inform the user about the acceptable file types.
- Testing a Website’s Login Page: Enter special characters or leave the password field blank to ensure the system does not allow login and prompts the user to enter a valid password.
- Testing an eCommerce Site’s Payment Gateway: Attempt to complete a purchase with an invalid credit card number or expired card and check if the system correctly rejects the payment and displays an error message.
- Testing an ATM Machine: Insert an invalid or expired bank card and try to withdraw money to confirm that the machine identifies the invalid card and denies the transaction with an appropriate error message.
- Testing a GPS Navigation System: Input a non-existent address or intentionally misspell a location to see if the system can handle the error and either offer suggestions or inform the user that the address is invalid.
- Testing a Mobile App’s Camera Function: Open the camera in the app and cover the lens entirely or try to take a picture when the phone’s storage is full to see if the app properly handles these scenarios and provides relevant feedback to the user.
When to Perform Negative Testing?
Negative testing should be performed at various stages of the software development process. It is especially important during the functional testing of the build where there are chances of unexpected conditions. By conducting negative testing early on, developers can identify and fix defects that might cause the application to fail. It’s also crucial to perform negative testing after every major change or update to the software, ensuring that no new issues have been introduced. This helps maintain the stability and reliability of the application.
How to Perform Negative Testing?
Negative testing is a method used to ensure that a software application can handle invalid input or unexpected user behavior gracefully. Here’s a simple guide to performing negative testing:
- Identify Test Cases: Determine the scenarios where users might input invalid data. This includes entering incorrect information in forms, special characters in text fields, or attempting actions without proper permissions.
- Prepare Invalid Data: Create a list of data that doesn’t meet the usual criteria. For example, this might be entering letters in a number field, leaving required fields blank, or using scripts to test for vulnerabilities.
- Execute the Test: Input the invalid data into your application. Check how the system reacts. Ideally, it should give an appropriate error message without crashing or malfunctioning.
- Document the Results: Record what happens when invalid data is entered. Note any errors encountered or unexpected behaviors.
- Review and Fix Issues: If the application does not handle the invalid data correctly, make necessary adjustments to the code. Ensure that proper validation and error handling mechanisms are in place.
By following these steps, you can ensure your application is robust and can handle unexpected situations effectively.
Techniques Used in Negative Testing
Techniques used for negative testing are:
Boundary Value Analysis:
It is related to the invalid partition in your input test data range. The system should reject the values for invalid inputs. Invalid partition is going to have 2 boundaries – lower and upper boundary. If the input test data range is A-B, negative test cases should be designed for A-1 and B+1.
Example #1: For date field (1-31), invalid partition lower boundary(input 0 in date field) and invalid upper boundary(input 32 in date field) are considered for negative test cases.
Example #2: For username fields having specifications of 6-10 characters, invalid partition lower boundary(5 characters) and invalid partition upper boundary(11 characters) are considered for negative test cases.
Example #3: For floating-point values, let the system accept values from 0.2 to 0.8 with one decimal place. Invalid partition lower boundary(input 0.1) and invalid upper boundary(input 0.9) are considered for negative test cases.
Read more about Boundary Value Analysis Test Case Design Technique here
Equivalence Partitioning:
In this technique, input test data is divided into partitions. For negative testing, if you pick a value from an invalid partition, the system should reject that value.
Example #1: For date fields (1-31), input any invalid value such as 0 and negative integer values, the system should reject the values.
Example #2: For username fields having specifications of 6-10 characters, input any value from invalid partition i.e. 0-5 or 11,12,13… and test the behavior of the system. In this case, the system should reject the values and display an error.
Example #3: For Age fields between 18-80 years except for 60-65 yrs, input any value from invalid partition i.e. 0-17 or 60-65 or 81,82,83… and test the behavior of the system.
Read more about Equivalence Test Case Design Technique here
Importance of Negative Testing
- Identifying Weaknesses: Helps to uncover hidden bugs that might not be found through normal testing methods.
- Ensuring Stability: Negative test cases assure that the software does not crash when faced with invalid or unexpected inputs.
- Preventing Data Corruption: Protects data integrity by checking how the system handles incorrect data.
- Enhancing Security: Reduces the risk of security vulnerabilities by stressing the software with unusual inputs.
- Improving Reliability: Negative tests make the software more dependable by ensuring it can handle unexpected situations smoothly.
- Boosting User Experience: Leads to a better user experience by preventing errors and ensuring smooth operation under all conditions.
Challenges of Negative Testing
Conducting negative testing can be challenging for several reasons.
- Time-Consuming: Requires significant time to create and execute tests for all possible invalid inputs.
- Complexity: Involves creating complex test scenarios which can be difficult to come up with.
- Resource Intensive: Needs additional resources such as tools, environments, and skilled testers.
- Maintenance: Keeping the negative test cases updated with changes in the software can be challenging.
- False Positives: Sometimes produces false positives, where a test fails due to incorrect test case design rather than a bug in the software.
- Limited Coverage: It is nearly impossible to anticipate and test for every possible negative scenario, leading to potential gaps.
Best Practices of Negative Testing
- Define Clear Objectives: Identify the main goals for negative testing. Know what you’re trying to achieve, such as improving security or ensuring stability.
- Cover Common Scenarios: Focus on scenarios that are most likely to occur. Think about how users might make mistakes or misuse the software.
- Use Equivalence Partitioning: Group similar invalid inputs together to simplify testing. This approach helps in covering a wide range of inputs without excessive redundancy.
- Automate Where Possible: Utilize automated testing tools to handle repetitive and time-consuming test cases. This saves time and reduces the chance of human error.
- Regularly Update Test Cases: Keep your test cases up-to-date with any changes in the software. Regularly review and adjust them as the software evolves.
- Validate Environment: Ensure that the testing environment closely mirrors the production environment. This ensures that the test results are realistic and applicable.
- Document Everything: Keep thorough documentation of your negative test cases, procedures, and results. This aids in understanding the test coverage and any issues encountered.
- Collaborate with Developers: Work closely with the development team to understand the software’s limits and use their insights to create more effective test cases.
- Prioritize Tests: Focus on the most critical areas first, such as security and critical functionalities, to ensure that key components are robust against negative scenarios.
- Perform Regular Reviews: Conduct regular reviews of your testing process and outcomes. Use feedback to continually improve your negative testing strategy.
Advantages and Disadvantages of Negative Testing
Advantages of Negative Testing
- Improves Software Quality: Negative testing helps identify weaknesses and vulnerabilities in the software, ensuring that it can handle unexpected situations gracefully.
- Increases Reliability: By simulating unusual or invalid inputs, negative testing ensures that the software remains stable and reliable under various conditions.
- Prevents Crashes: It helps in discovering potential crash scenarios before they impact real users, reducing the risk of software failure.
- Enhances Security: Negative testing can reveal security loopholes that attackers might exploit, helping to safeguard sensitive data.
- Better User Experience: By ensuring that the software can handle errors smoothly, it provides a more seamless and robust user experience.
Disadvantages of Negative Testing
- Resource-Intensive: Negative testing can be time-consuming and require a lot of resources, including specialized tools and environments.
- Complex to Execute: Setting up tests for all possible invalid inputs can be complex and requires a high level of expertise.
- Difficult to Predict: Foreseeing every way a user might misuse the software is challenging, making it hard to cover all negative scenarios.
- Interpreting Results: Understanding why the software fails and determining whether it behaved correctly under negative testing can be difficult and require detailed analysis.
- High Costs: The need for specialized tools and environments can increase the cost of testing, especially for small teams or projects with limited budgets.
What is the difference between Positive Testing & Negative Testing
Positive Testing | Negative Testing |
---|---|
It is performed by passing valid test data | It is performed by passing invalid test data |
It is performed to verify the known set of Test Conditions | It is performed to break the application with unknown set of Test Conditions |
It covers only valid cases | It covers all possible cases including invalid cases |
It takes less time | It takes more time |
It verifies all the requirements are met | It verifies the work flows which are not mentioned in the requirements |
It makes sure software is working as expected | It makes sure the software is defect free |
Conclusion
Negative testing is crucial as it helps identify potential points of failure that may not be discovered during positive testing, where valid input and expected conditions are tested. By addressing these issues, the software becomes more robust and user-friendly.
Related posts:
- Software Testing Tutorial (Free Software Testing Course)
- Manual Testing Interview Questions
- 100+ Types of Software Testing
- Software Development Life Cycle (SDLC) – Complete Guide
- Software Testing Life Cycle (STLC) – Complete Guide
- Bug Life Cycle (Defect Life Cycle) – Complete Guide
- When and how we do Regression Testing in Software Development
- When we do Retesting in Software Development
- Software Testing Tools
- Prompt Engineering In Software Testing
- How To Become A Prompt Engineer (Step By Step Guide)
- 30+ Prompt Engineering Interview Questions
- Artificial Intelligence In Software Testing
- What is AI Testing | Everything You Should Know
- Know about International Tester’s Day