40+ Python Interview Questions & Answers
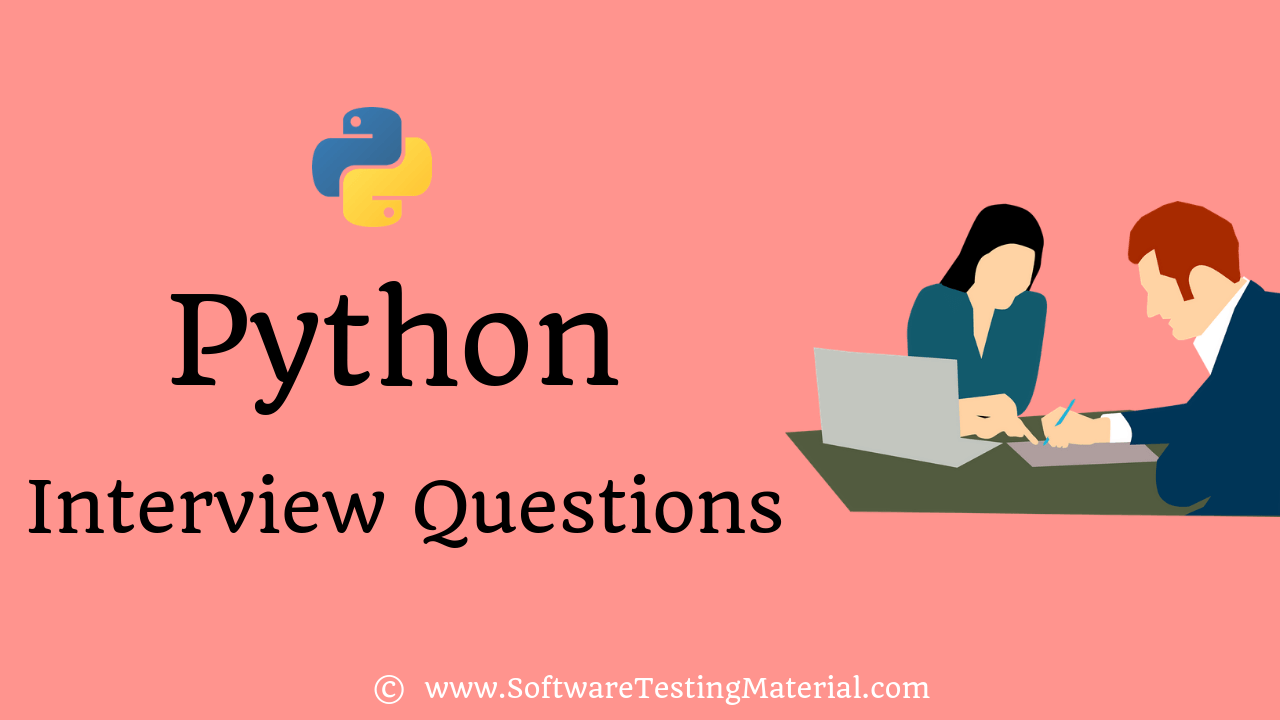
Here we are going to cover most frequently asked Python interview questions and answers on Python programming language. Hopefully, this list of interview questions will be the one-stop resource in your search on Python coding interview questions which helps you to crack any Python interview.
Experienced Python programmers might quickly catch up with the competition than those who recently started studying Python programming. So here we have compiled a list of Python programming interview questions for both beginners and experienced.
Also read: Java Interview Questions
Let’s now step-in to explore the Python Q&A section. However, it’s entirely possible that you already know some of them. But we think you would still benefit from reading.
A list of frequently asked Python interview questions with answers for freshers and experienced are given below.
Basic Python Interview Questions 1 – 25
1. What is Python? What are the benefits of using Python?
Python is an open-source interpreted, high-level, object-oriented scripting language. It was created by Dutch programmer Guido van Rossum, and released in 1991.
- Application development is faster and easy
- It is one of the fastest-growing programming languages in the world
- Its easy to keep the code base readable and application maintainable due to its simple easy-to-use syntax rules
- It doesn’t require explicit memory management as the interpreter itself allocates the memory to new variables and free them automatically.
- It can be used for server-side web development, software development, mathematics, artificial intelligence, data analysis, data science, system scripting, scientific computing etc.,
- It comprises of a huge standard library which includes areas like internet protocols, string operations, web services tools and operating system interfaces. Many high use programming tasks have already been scripted into the standard library which reduces length of code to be written significantly.
- It has a massive support community to get your answers. Thanks to the fact that it is open source and community developed.
2. Features of Python?
- Easy to learn and use
- Expressive language
- Interpreted language
- Cross-platform language
- High level language
- Portable
- Free and open source language
- Object oriented language
- Extensible
- Large standard library
- GUI Programming support
- Integrated
- Embeddable
- Dynamically Typed Language
3. What is PEP 8?
PEP stands for Python Enhancement Proposal. PEP 8 is Python’s style guide. It’s a set of rules for how to write your Python code more readable.
4. How is Python script executed ?
When a Python script is executed, it doesn’t convert its code into machine code. It actually converts it into something called byte code. Execution of Python script means execution of the byte code on the Python Virtual Machine (PVM).
Suppose your script is in “D:\myPythonCode\script.py”
— Open Command line: Start menu -> Run and type cmd
— Type: C:\python27\python.exe D:\myPythonCode\script.py
5. What is the difference between .py and .pyc files ?
.py files are the original text files holding Python code.
.pyc files are the compiled version of the .py files. These are byte code files that is generated by the Python compiler.
Python compiles the .py files and saves it as .pyc files
6. Is Python case sensitive?
Yes. Python is a case sensitive language.
7. What is pickling and unpickling in Python?
Pickling:
Pickling is a process in which pickle module accepts any Python object and converts it into a string representation and dumps the same into a file by calling the dump method.
Unpickling:
Unpickling is a process in which retrieving original Python objects from a stored string representation. Simply, the reverse process of pickling is known as unpickling.
8. What is monkey patching in Python?
Monkey Patching in Python refers to the dynamic modifications made to a class or module at runtime
9. What are python modules? Name some commonly used built-in modules in Python?
Python modules are files consisting of Python code. A Python module can define functions, classes and variables. A Python module is a .py file containing runnable code.
10. What are local and global variables in Python?
Local variables:
Variables declared inside a function is known as a local variable. These variables are present in the local space and not in the global space. locals() is accessed within the function and it returns all names that can be accessed locally from that function.
Global variables:
Variables declared outside a function or in global space are called global variables. These variables can be accessed by any function in the program. globals() returns all names that can be accessed globally from that function.
11. What is the difference between a list and a tuple in Python?
List vs Tuple
List:
- Lists are mutable. A mutable data type means that a python object of this type can be modified
- Lists is a sequence of elements which are separated by commas and are surrounded by a square brackets or simply brackets “[]”.
- List has variable length
- Lists operation has more size than that of tuple, which makes it a bit slower
Tupple:
- Tuples are immutable. An immutable objects can’t be modified. We can’t modify a tuple object after it’s created
- Tuples is a sequence of elements which are separated by commas and are surrounded by a round brackets or parentheses “()”
- Tuple has fixed length
- Tuples operation has smaller size than that of list, which makes it a bit faster
12. What does len() do?
It is used to determine the length of a string, a list, an array, etc.
a=’STM’
len(a)
13. What are *args and **kwargs in Python?
In Python, *args and **kwards are used to pass a variable number of arguments to a function using special symbols.
We use *args and **kwargs as an argument when we aren’t sure how many arguments to pass in the functions.
14. How is Python an interpreted language?
Python is an interpreted language. Python program runs directly from the source code. It converts the source code that is written by the programmer into an intermediate language, which is again translated into machine language that has to be executed. It converts the source code into an intermediate language, which is again translated into native language / machine language that has to be executed.
15. What are the built-in types in Python?
Common native data types in Python are as follows.
1. Mutable built-in types: We can change the content without changing the identity
- Dictionaries
- List
- Sets
2. Immutable built-in types: We can’t change the content once it is created
- Strings
- Tuples
- Numbers
16. How memory is managed in Python?
- Memory in Python is managed by Python private heap space. All Python objects and data structres are located in a private heap. The programmer doesn’t have an access to the private heap and this private heap in Python is taken care by Python Interpreter.
- Python memory manager is responsible in allocating Python heap space for Python objects.
- Python has an inbuilt garbage collector, which recycles all the unused memory and frees the memory and makes it available to the heap space.
17. What are the tools that help to find bugs or perform static analysis?
PyChecker: PyChecker is a static analysis tool that finds bugs in the Python source code and raises alerts about the issues in the style or code complexity.
Pylint: Pylint checks if a module meets the coding standards.
18. What are Python decorators?
Python decorators allow programmers to add functionality to an existing code. It is to wrap a function in order to extend the behavior of wrapped function, without permanently modifying it.
19. What are the different environment variables identified by Python?
Environment variables, which can be recognized by Python are
- PYTHONPATH
- PYTHONSTARTUP
- PYTHONCASEOK
- PYTHONHOME
20. What is PYTHONPATH?
PYTHONPATH is an environmental variable identified by Python which tells the interpreter where to locate the module files imported into a program.
21. What is PYTHONSTARTUP?
PYTHONSTARTUP is an environmental variable identified by Python which contains the path of an initialization file containing Python source code.
22. What is PYTHONCASEOK?
PYTHONCASEOK is an environmental variable identified by Python which is used in windows to instruct Python to find the first case-insensitive match in an import statement
23. What is PYTHONHOME?
PYTHONHOME is an environmental variable identified by Python which is an alternative module search path. Usually it is embedded in the PYTHONSTARTUP or PYTHONPATH directories to make switching module libraries easy.
24. What is a Name in Python?
A Name in Python is also known as Identifier. It is a way to access a variable. It is simply a name given to the objects. In Python, we can declare a variable by just assigning a name to it.
25. What is the Namespace in Python?
A Namespace is a naming system used to ensure the names are unique for each and every object (might be a variable or a method) in Python to avoid naming conflicts. Based on the namespace, Python interpreter understands what exact method or variable one is trying to point to in the code
Basic Python Interview Questions for data science:
26. What is a scope in Python?
We may not be able to access all of the namespaces from every part of the program. Scope refers to the coding region from where a namespace can be accessed.
27. What are the iterators in Python?
In Python, iterators are used to iterate containers like a list or a group of elements. Iterator implements __itr__ and next() method to iterate the stored elements. In Python, we generally use loops to iterate over the collections (list, tuple).
28. What is slicing in Python?
Slicing is a string operation used to select a range of items from sequence type like list, tuple, and string.
The slice object represents the indices specified by range(start, stop, step). The slice() method allows three parameters ie., start, stop, and step.
start – starting number for the slicing to begin.
stop – the number which indicates the end of slicing.
step – the value to increment after each index (default = 1).
Although we can get elements by specifying an index. In Python, a string (say text) begins at index ‘0’, and the nth character stores at ‘n-1’. We can also do reverse indexing using negative numbers. By doing this we can get only single element whereas using slicing we can get a group of elements. It is beneficial and easy to get elements from a range by using slice.
29. What is a dictionary in Python?
Dictionary in python is a collection which is unordered, changeable and indexed. Dictionaries are written with curly brackets, and the elements in python dictionaries are stored as key-value. Dictionaries are indexed by keys and are optimized to retrieve values when the key is known. The keys are unique whereas values can be duplicate.
30. What is Pass in Python?
Pass statement is a null operation. Nothing happens when it executes. Pass statement helps to pass the control without an error when we want to create an empty class or function.
Example:
If(x>100)
print(“Python Interview Questions”)
else
pass
31. What statement is used in Python if the statement is required syntactically but there is no execution of code or command?
We use Pass statement.
32. What is the command to debug a Python program?
The command used to run a Python program in debug mode is
$ python -m pdb python-script-file-name.py
33. What are parameters and arguments in python?
A parameter is a variable which is passed during the declaration of a function.
An argument is the actual value of the variable (parameter) that gets passed to the function. When a function is called, the arguments are the actual value we pass into the function’s parameters.
// x and y are parameters
// function definition
def add(x,y):
return x+y
// 10 and 20 are arguments
// function call
result=add(10,20)
print(result)
34. Are arguments pass by value or pass by reference in Python?
Python neither passes by value nor by reference. Python passes by ‘Assignment‘. Everything in python is an object. The parameter we pass is originally a reference to the object not the reference to a fixed memory location. We cannot change the value of the references but we can change the objects if it is mutable.
35. What’s the Difference between a List and a Dictionary?
List vs Dictionary:
List:
- A Python List can be used to store a sequence of elements that are mutable. So the elements can be modified after they are created. They can be of any data type. They can all be the same or they can be mixed.
- Elements in lists are always accessed through numeric, zero-based indices.
- Lists can be used whenever you have a collection of items in an order
Dictionary:
- A Python dictionary is an unordered collection of key-value pairs. Dictionaries are indexed by keys and are optimized to retrieve values when the key is known. The keys are unique whereas values can be duplicate.
- Elements in the dictionary can be accessed by using their key.
- A dictionary can be used whenever you have a set of unique keys that map to values.
36. How would you get a list of all the Keys in a Dictionary?
To get a list of all the keys in a dictionary, I use function keys():
mydict={‘x’:90,’y’:91,’z’:92}
mydict.keys()
dict_keys([‘x’, ‘y’, ‘z’])
37. When Would You Use a List vs. a Tuple vs. a Set in Python?
A Python List can be used to store a sequence of elements that are mutable. So the elements can be modified after they are created. They can be of any data type. They can all be the same or they can be mixed. It is ideal for projects that demand the storage of objects that can be changed later.
Tuples are immutable. An immutable objects can’t be modified. We can’t modify a tuple object after it’s created. Tuples operation has smaller size than that of list, which makes it a bit faster. It is ideal for projects that demand a list of constants.
A Python Set can be used to store a collection of unique elements. It is ideal for projects that demand no duplicate elements in a list. If we have two lists with common elements between them then we can leverage sets to eliminate them.
38. How to remove duplicate elements from the list in Python?
There are different ways to remove duplicate elements from the list. One of the methods is to iterate over the list and identify duplicates and remove them. The most popular way to remove duplicate elements from the list is using Sets.
a = [1,4,4,5]
list(set(a))
39. What is lambda in Python?
A lambda function is a small anonymous function in Python. It can take any number of arguments, but can only have one expression.
To define a lambda function, first write keyword lambda (instead of def) followed by one of more arguments separated by comma, followed by colon sign (:), followed by a single line expression.
Syntax:
lambda arguments : expression
Example:
a = lambda x, y: x * y
a(4, 3) # Call the lambda function
Output: 12
40. What is the difference between Lambda function and normal function (def function) in Python?
Lambda function Vs. Def function.
- Lambda contains only one expression and can accept any number of arguments whereas Def can hold multiple expressions
- Lambda returns a function object which can be assigned to any variable whereas a Def function contains a function name, pass the parameter and mandatorily have a return statement
- Lambda can’t have return statements whereas Def can have a return statement
41. What is unittest in Python?
Unittest in Python is a unit testing framework. Unit testing is the first level of Software Testing. In Unit testing, we test the smallest testable parts to validate each unit of the software performs as expected.
It supports the following:
- Sharing of setups
- Automation testing
- Aggregation of tests into collections
42. How can you copy an object in Python?
There are two ways to create copies in Python
- Shallow Copy (copy.copy())
- Deep Copy (copy.deepcopy())
43. What is the difference between deep copy and shallow copy?
Deep copy:
- It doesn’t copy the reference to the objects. It means changes made to a copy of object do not reflect in the original object.
- It is implemented using “deepcopy()” function.
- It makes execution of a program slower compared to shallow copy.
Shallow copy:
- It copies the reference to the objects. It means changes made to a copy of object do reflect in the original object.
- It is implemented using “copy()” function.
- It makes execution of a program faster compared to deep copy.
44. How you can convert an int to a string?
Python has a built-in function str() for converting an integer number to string. We need to pass an integer number to this function. It will convert the integer to a string.
45. How to convert an integer number to octal string in Python
Python has a built-in function oct() for converting an integer number to octal. We need to pass an integer number to this function. It will convert the integer to octal string and returns the value.
This function returns a octal string prefixed with ‘0o’
46. How to convert an integer number to hex string in Python
Python has a built-in function hex() for converting an integer number to hexadecimal string. We need to pass an integer number to this function. It will convert the integer to hexadecimal string and returns the value.
This function returns a lowercase hexadecimal string prefixed with ‘0x’
47. What are control flow statements in Python?
Usually the flow of execution of a program runs from top-down order in Python. To break this top-down order and manipulate the execution flow of a program, we use control flow statements.
These control flow statements are used whenever a program should take decision depends on the situation such as displaying ‘Good morning’ or ‘Good night’ depending on the time.
There are three control flow statements in Python – if, for and while.
Conclusion
We hope this set of Python programming Interview Questions helped you in preparing for your Python interview.
If you are a Software Tester, it helps you too. This post covered python interview questions for QA testers too. It helps you to clear your Selenium with Python Interview.
Got a question for us? Please mention it in the comments section and we will get back to you at the earliest.
Get this python interview questions and answers for experienced pdf download
Here are some top choices for Python books to double-check your Python preparation.
Also Read:
- Python Strings
- Python Data Types
- Python Multiline String
- Python Join() Method with Examples
- Python float() Function Explained with Examples
- Python Interview Questions
- How to Convert Python List to String (4 Ways)
Nice sir and it’s very usefull sir actually I belongs to non IT batch sir it’s very helpful for me and I am thanking you sir
Thanks. Please share with your friends too.