Protractor Testing Tutorial – End-To-End Testing of AngularJS Applications
In this Protractor Testing Tutorial you will learn the following
- What is Protractor
- Features of Protractor
- What is Selenium
- Difference between Selenium and Protractor
- Which Framework to use
- Protractor Installation
- Creating First Protractor Test Case
- Execution of the Code
- Generate Reports using Protractor Beautiful Report
- Known issues with Protractor installation
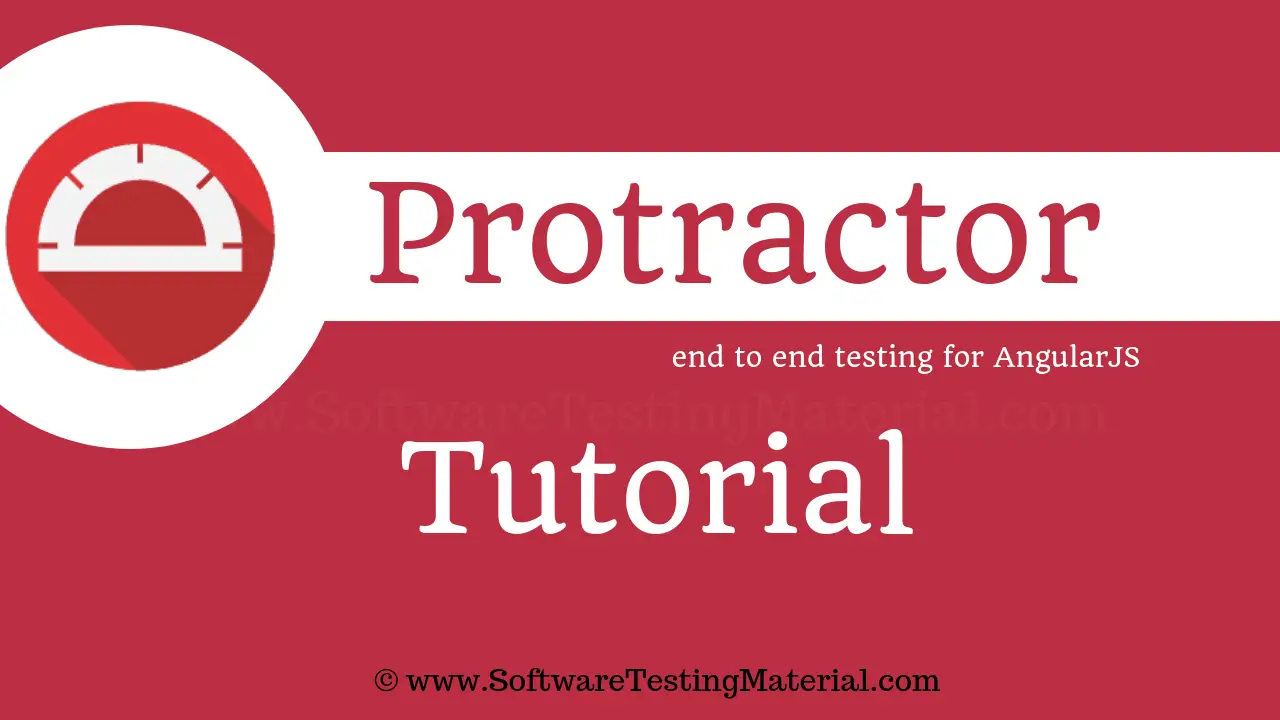
What is Protractor?
Protractor is an end-to-end test framework for Angular and AngularJS applications. It is designed by Google Developers to support AngularJS applications in mind. It is released as an open source framework. This behavior-driven test framework is intended not only to test AngularJS applications but also for non-AngularJS applications as well. It combines the powerful technologies like Selenium, WebDriver, Jasmine, Node.js etc., It runs tests against your application running in a real browser, interacting with it as a user would. It is a node.js port of the webdriver.io, which is the JavaScript implementation of Selenium framework. It is a Node.js program that supports test frameworks like Jasmine, Mocha, and Cucumber.
It is built on top of WebDriverJS. It supports angular specific features and also all the features which are supported by Selenium WebDriver. It supports Angular-specific locator strategies, which allows you to test Angular-specific elements without any setup effort on your part.
Must Read: Protractor Interview Questions
Features of Protractor
- It Supports both Angular and Non-Angular applications – No matter whether your application is Angular or Non-angular, protractor supports it.
- It supports angular specific locators. The angular applications come with angular specific locators such as ng-modal, ng-repeat etc., There is no point to create complex XPath for angular locators when using Protractor. Protractor has extended support for angular specific locators. You can use by.model, by.repeater etc., to handle these angular specific locators.
- It supports parallel-execution
- It supports cross-browser testing
- It supports mobile browsers too. We can run the same script in mobile browser too without changing the code.
- It supports Headless browser
- It supports cloud testing platforms such as crossbrowsertesting.com and SauceLabs
- It supports CI/CD tools such as Jenkins, TFS etc.,
- It supports for Behavior-driven development such as Jasmine/Mocha
- We can deal easily with synchronization issue in AngularJS applications
- Protractor uses a control flow mechanism internally and it makes sure that commands are executed in the order they were received. Even though most webdriver actions are asynchronous, there is no need to worry about asynchronous behaviour of the application.
What is Selenium?
Selenium is one of the automation testing tools which is an open source tool. Selenium automates web application. You can check complete Selenium WebDriver Tutorial here and also go through this most important Selenium Interview Questions
Difference between Selenium and Protractor?
Selenium:
- Selenium supports languages such as Java, Python Kotlin, C, C#, PHP.
- Selenium supports frameworks such as TestNg and Junit
- Performance wise selenium is faster when working with non-Angular applications
- It is an open source software
- Java and TestNG are necessary to work with Selenium
- Compatible on Windows and Linux.
- You can automate both Angular and Non-Angular pages too but you have to overcome synchronization issues.
- Angular JS applications have some extra HTML attributes like ng-repeater, ng-controller, ng-model, etc. which are not included in Selenium locators. So, it is difficult to capture the web elements in AngularJS applications using Selenium.
Protractor:
- Protractor supports all js related languages such as Type Script and Java Script
- It supports frameworks such as Jasmine and Mocha
- Performance wise protractor is faster when working with Angular applications
- It is also an open source software
- JavaScript and Node.js are necessary to work with Protractor
- Compatible on Windows and Linux.
- It is specially designed to support AngularJs applications but you can automate both Angular and Non-Angular pages.
- Protractor supports Angular-specific locator strategies. So, it is easy to capture the web elements in AngularJS applications using Protractor.
Which Framework to use?
It supports two Behavior-driven development (BDD) test frameworks such as Jasmine & Mocha.
Jasmine:
Jasmine is an open source testing framework for JavaScript. It aims to run on any JavaScript-enabled platform. It doesn’t require a DOM and it has a clean, obvious syntax so that we can easily write tests.
Mocha:
Mocha is a JavaScript test framework for Node.js programs, featuring browser support, asynchronous testing, test coverage reports, and use of any assertion library.
How to Set Up Protractor (Protractor Installation)
Let’s see how to install Protractor. To setup Protractor, we need to follow the following steps.
- Install Node.js
- Install Protractor
- Update WebDriver Manager to the latest version
To successfully install protractor, check our step by step Protractor Installation guide with screenshots.
Creating First Protractor Test Case by using sample AngularJS application
Protractor needs the following two files to run
- Configuration file
- Spec file
Configuration file
The configuration file tells Protractor how to set up the Selenium Server, which tests to run, how to set up the browsers, and which test framework to use. The configuration file can also include one or more global settings. The config file provides explanations for all of the Protractor configuration options. Default settings include the standalone Selenium Server, the Chrome browser, and the Jasmine test framework. If any configuration is not defined in configuration files, it will use defaults.
Spec file
Spec file is the one where we write actual test code. It contains the logic and locators to interact with an application.
Open https://angularjs.org and pass the text as “Software Testing Material” in “Enter a name here” textbox.
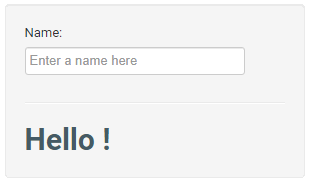
It shows the output text as “Hello Software Testing Material“
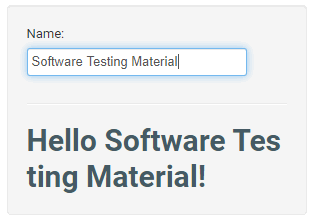
Let’s take a sample test case that navigates to a specific URL and checks its page title. To do that we have to follow below steps
Open a new command line and create a clean folder for testing.
Create configuration file (conf.js) and spec file (spec.js)
Copy the following into spec.js:
//Spec.js describe('Protractor Demo Application', function() { it('to check the page title', function() { browser.ignoreSynchronization = true; browser.get('https://www.softwaretestingmaterial.com/'); browser.driver.getTitle().then(function(pageTitle) { expect(pageTitle).toEqual('Software Testing Material'); }); }); });
Code Explanation of spec.js:
describe(‘Protractor Demo Application’, function()
The describe syntax is from the Jasmine framework. “describe” (‘Protractor Demo Application’) defines components of an application, which can be a class or function etc., and “Protractor Demo Application” is a string.
it syntax is from the Jasmine framework. it may contain some of test scenarios/steps etc., Based on your requirement, you can add multiple it blocks in your program.
browser.ignoreSynchronization = true;
SoftwareTestingMaterial.com is a non-angular website. So we set the ignoreSynchronizationtag to ‘true’.
browser.get(‘https://www.softwaretestingmaterial.com/’);
browser is a global created by Protractor, which is used for browser-level commands such as browser.ignoreSynchronization, browser.get.
expect(pageTitle).toEqual(‘Software Testing Material’);
‘expect‘ is an assertion where we are verifying the text which we have got from the webpage (pageTitle) with expected text (Software Testing Material)
Now create the configuration file.
Copy the following into conf.js:
// conf.js exports.config = { framework: 'jasmine', seleniumAddress: 'http://localhost:4444/wd/hub', capabilities: { browserName: 'chrome', }, specs: ['spec.js'] };
Code Explanation of conf.js:
This configuration tells Protractor the core details such as where your test files (specs) are, and where to talk to your Selenium Server (seleniumAddress).
framework: ‘jasmine’,
It specifies that we are using the test framework ‘Jasmine’.
Browser configuration is set inside the capabilities section. We can also specify other browsers such as firefox.
Chrome is the default browser and uses the defaults for all other configuration.
specs: [‘spec.js’]
The above code tells protractor the location of the test files. The path of the spec file is given in the ‘specs’ section
Execution of the Code
Make sure selenium web driver manager is up and running. Open the command prompt and run the command as “webdriver-manager start“
Now run the test with “protractor conf.js” in a new command prompt window
Protractor will execute the configuration file with given spec file in it.
You can notice that Selenium server running at “http://localhost:4444/wd/hub” which we have given in the conf.js file. You should see a Chrome browser window open up and navigate to SoftwareTestingMaterial.com website.
The test output should be 1 spec, 0 failure.
Let’s see an example test case which runs on AngularJS webpage. So let’s modify the spec.js file
// spec.js describe('Protractor Demo Application', function() { it('should multiply two integers', function() { browser.get('http://juliemr.github.io/protractor-demo/'); element(by.model('first')).sendKeys(2); element(by.model('second')).sendKeys(7); element(by.model('operator')).click(); element(by.xpath(".//option[@value= 'MULTIPLICATION']")).click(); element(by.id('gobutton')).click(); expect(element(by.binding('latest')).getText()).toEqual('21'); //Incorrect expectation //expect(element(by.binding('latest')).getText()).toEqual('14'); //Correct expectation }); });
With the above code, you can see what exactly Protractor is capable of. There is no browser synchronization code in the above spec.js
Run this file same like we ran earlier one.
protractor conf.js
A web page will open which contains a calculator.
Here we have used the globals element and by, which are also created by Protractor. The element function is used to find HTML elements on a webpage.
sendKeys() is to pass values in the text boxes.
click() is used to click a button
getText() to return the content of an element
Here in the above code, we have used Angular elements such as ‘by.model’ which locates angular elements.
element() describes how to find the element. It takes one parameter.
by() is an object which creates locators.
In the above code, we used three types of locators.
- by.model(‘first’) – it is to find the element with ng-model=”first”. If you inspect the calculator page source you can find the following
<input type=”text” ng-model=”first”> - by.id(‘gobutton’) – it is to find the element with the given id. This finds <button id=”gobutton”>.
- by.binding(‘latest’) – it is to find the element bound to the variable latest. This finds the span containing {{latest}}
Learn more about locators and ElementFinders.
You should see a Chrome browser window open up and navigate to the Calculator, then close itself. You can see the result say how many are passed and how many are failed.
You can see it passes two numbers and wait for the result to be displayed. Because the result is 21, not 14, our test fails.
The test output should be 1 spec, 1 failure.
Fix the test and try running it again. If you pass the value as 14 then you can see the test out put as 1 spec, 0 failure
Conclusion:
We can use Selenium to work on AngularJS applications by identifying the web elementsusing XPath or CSS selector. The web elements in the AngularJS applications will be generated and changed dynamically. To overcome this situation, it is better to use Protractor to work with AngularJS applications to make your life super easier and also it is a best practice to test AngularJS applications.
Generate Reports using Protractor Beautiful Report:
We can generate Protractor HTML reports. We have published a detailed post Protractor Beautiful Reports which deals with HTML Reports, Screenshots & Logs.
Known issues with Protractor installation:
If you face an errors such as “Unhandled error” or “Protractor Selenium standalone has exited with code 1” while installing protractor in global mode, then try the following solutions.
Solution 1: Open command prompt and run
webdriver-manager clean
Above code is to clear all the downloaded binaries
webdriver-manager update
Above line is to reinstall the binaries.
Solution 2:
Install webdriver manually.
Download the Selenium jar manually and paste the .jar in the below folder:
C:\Users\User_Name\AppData\Roaming\npm\node_modules\protractor\selenium
Must read: