Selenium 4 | How To Capture A Full-Page Screenshot Using Selenium 4
In this post, we will see Selenium 4 screenshot features. Using Selenium 3, we can capture screenshot of a web page.
To capture a full page screenshot in Selenium 3, we used to use aShot WebDriver Screenshot utility.
By using Selenium WebDriver 4’s screenshot feature, we can capture screenshot at the element, section, and full-page level.
Don’t miss: New features in Selenium 4
How to take screenshots in Selenium 4?
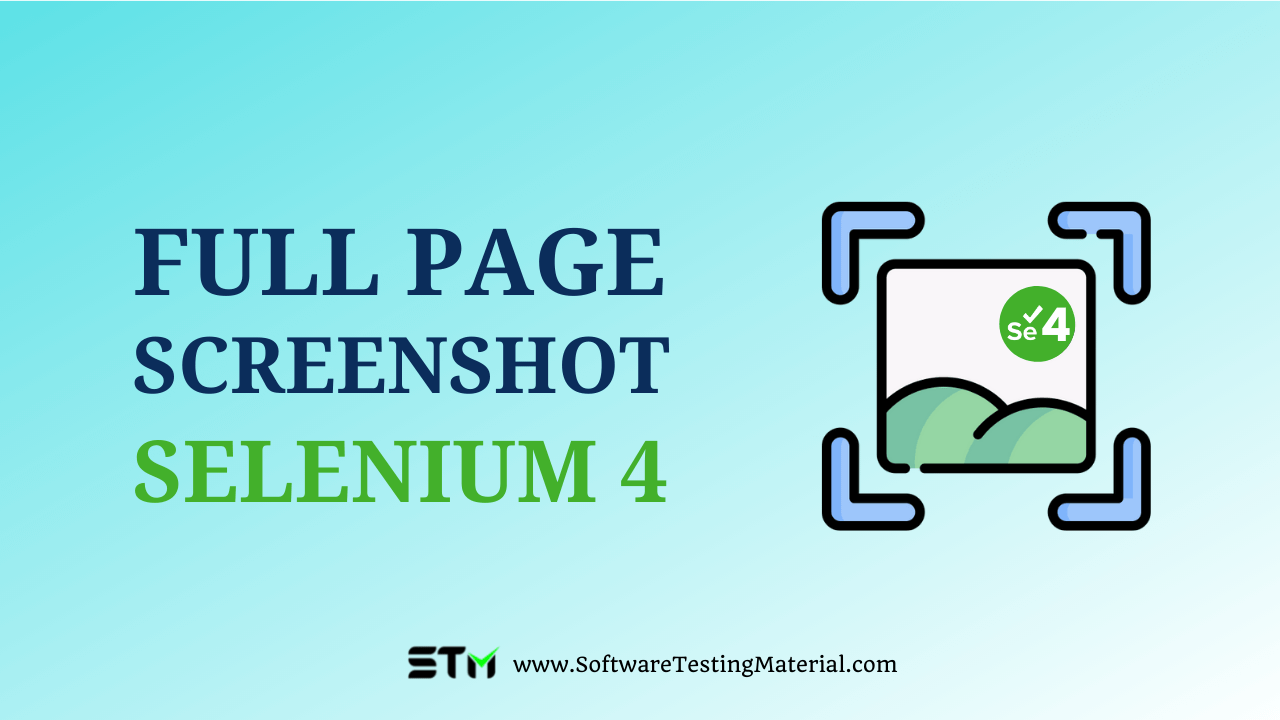
As discussed above we can take screenshots in element level, section level, and full-page level using Selenium 4. Let’s see each in detail along with sample code.
#1. Element Level Screenshot
In Selenium 4, there is a new method called getScreenshotAs(). It allows us to take a screenshot of a particular WebElement with this method.
Following code allows you to take a screenshot of the “STM logo” on “SoftwareTestingMaterial” homepage.
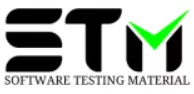
package stm.test; import org.testng.annotations.BeforeClass; import org.testng.annotations.AfterClass; import org.testng.annotations.Test; import org.openqa.selenium.By; import org.openqa.selenium.JavascriptExecutor; import org.openqa.selenium.OutputType; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.firefox.FirefoxDriver; import org.openqa.selenium.io.FileHandler; import org.openqa.selenium.support.ui.ExpectedConditions; import org.openqa.selenium.support.ui.WebDriverWait; import io.github.bonigarcia.wdm.WebDriverManager; import java.io.File; import java.io.IOException; import java.time.Duration; public class ElementSectionScreenshot { public WebDriver driver; @BeforeClass public void setUp() { WebDriverManager.firefoxdriver().setup(); driver = new FirefoxDriver(); driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(10)); driver.get("https://www.softwaretestingmaterial.com"); } @AfterClass public void afterClass() { driver.quit(); } @Test public void captureWebElementScreenshot() { WebElement elementLogo = driver.findElement(By.className("has-logo-image")); File src = elementLogo.getScreenshotAs(OutputType.FILE); File dest = new File(System.getProperty("user.dir") + "/screenshots/elementLogo.png"); try { FileHandler.copy(src, dest); } catch (IOException exception) { exception.printStackTrace(); } } }
#2. Section Level Screenshot
On the STM Homepage, there is a menu in the top right hand side as shown below.

Following code allows you to take a screenshot of the menu on “SoftwareTestingMaterial” homepage.
package stm.test; import org.testng.annotations.BeforeClass; import org.testng.annotations.AfterClass; import org.testng.annotations.Test; import org.openqa.selenium.By; import org.openqa.selenium.JavascriptExecutor; import org.openqa.selenium.OutputType; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.firefox.FirefoxDriver; import org.openqa.selenium.io.FileHandler; import org.openqa.selenium.support.ui.ExpectedConditions; import org.openqa.selenium.support.ui.WebDriverWait; import io.github.bonigarcia.wdm.WebDriverManager; import java.io.File; import java.io.IOException; import java.time.Duration; public class ElementSectionScreenshot { public WebDriver driver; @BeforeClass public void setUp() { WebDriverManager.firefoxdriver().setup(); driver = new FirefoxDriver(); driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(10)); driver.get("https://www.softwaretestingmaterial.com"); } @AfterClass public void afterClass() { driver.quit(); } @Test public void captureSectionScreenshot() throws InterruptedException { WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10)); wait.until(ExpectedConditions.visibilityOfElementLocated(By.className("site-header-main-section-right"))); WebElement elementLogo = driver.findElement(By.className("site-header-main-section-right")); File src = elementLogo.getScreenshotAs(OutputType.FILE); File dest = new File(System.getProperty("user.dir") + "/screenshots/sectionTutorials.png"); try { FileHandler.copy(src, dest); } catch (IOException exception) { exception.printStackTrace(); } } }
#3. Full-Page Screenshot
Let’s see How to take a screenshot of the full page in Selenium.
In Selenium 4, there is a method called getFullPageScreenshotAs() for Firefox. It allows us to take a full page screenshots and store it in the specified location.
Here, instead of typecasting it to the ‘TakeScreenshot‘ interface. We have to typecast it to the ‘FirefoxDriver‘ instance. Since it works only on Firefox browser.
File src = ((FirefoxDriver) driver).getFullPageScreenshotAs(OutputType.FILE);
Following code allows you to take a full-page screenshot of the “SoftwareTestingMaterial” homepage.
package stm.test; import java.io.File; import java.io.IOException; import java.time.Duration; import org.openqa.selenium.OutputType; import org.openqa.selenium.WebDriver; import org.openqa.selenium.firefox.FirefoxDriver; import org.openqa.selenium.TakesScreenshot; import org.openqa.selenium.io.FileHandler; import org.testng.annotations.AfterClass; import org.testng.annotations.BeforeClass; import org.testng.annotations.Test; import io.github.bonigarcia.wdm.WebDriverManager; public class FullPageScreenshot { public WebDriver driver; @BeforeClass public void setUp() { WebDriverManager.firefoxdriver().setup(); driver = new FirefoxDriver(); driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(10)); driver.get("https://www.softwaretestingmaterial.com/category/tutorial/"); } @AfterClass public void afterClass() { driver.quit(); } @Test public void captureFullPageScreenshot() throws IOException { File src = ((FirefoxDriver)driver).getFullPageScreenshotAs(OutputType.FILE); FileHandler.copy(src, new File("FullPageScreenshot.png")); } @Test public void capturePageScreenshot() throws IOException { /*TakesScreenshot interface enables the Selenium WebDriver to take a screenshot and store it in different ways. "getScreenshotAs()" method captures the screenshot and store it in the specified location.*/ File src = ((TakesScreenshot) driver).getScreenshotAs(OutputType.FILE); FileHandler.copy(src, new File("PageScreenshot.png")); } }
The above code captures two screenshots
- FullPageScreenshot.png
- PageScreenshot.png
1. FullPageScreenshot.png – Full webpage of SoftwareTestingMaterial website from top to bottom.
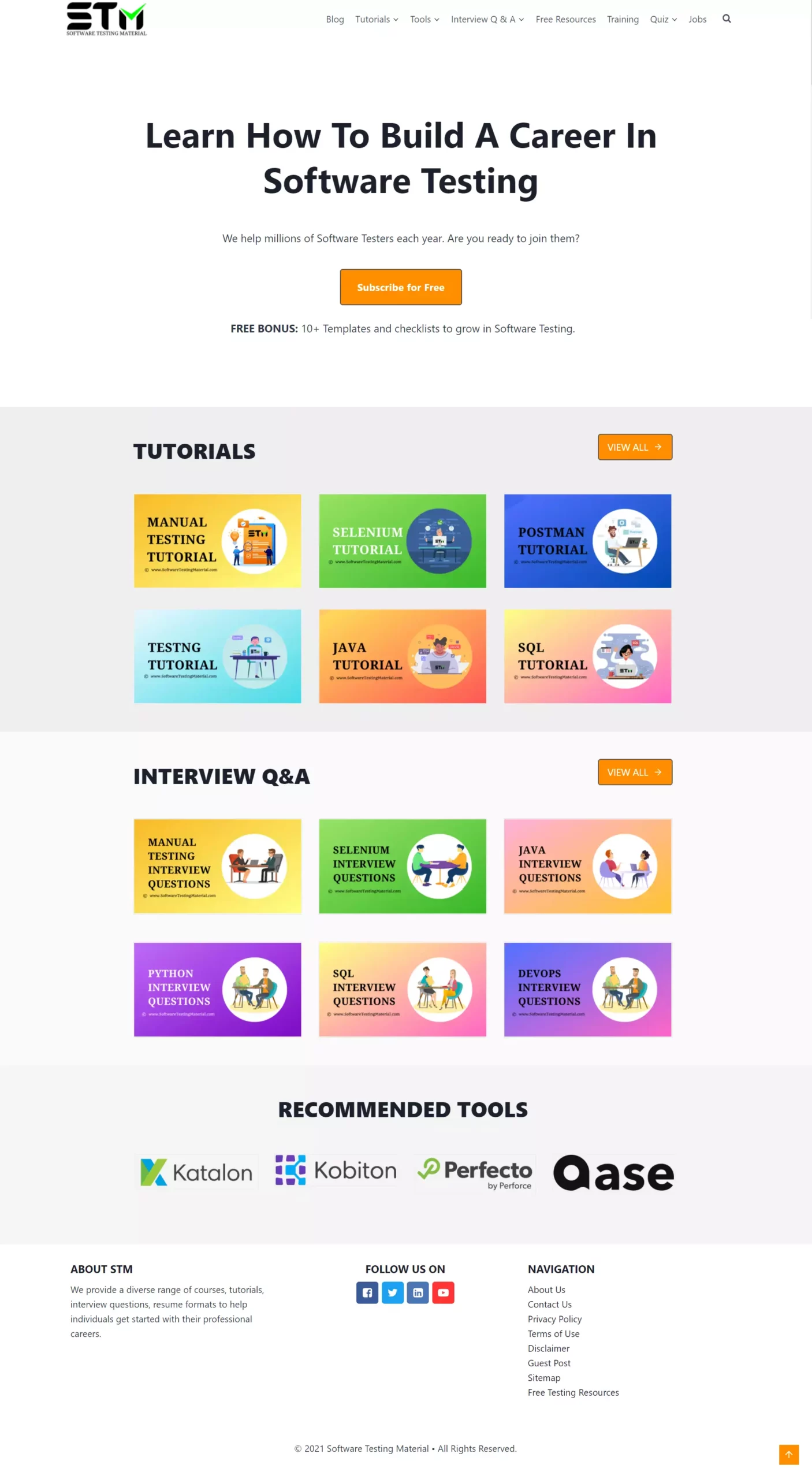
2. PageScreenshot.png – Only visible part of the SoftwareTestingMaterial website.
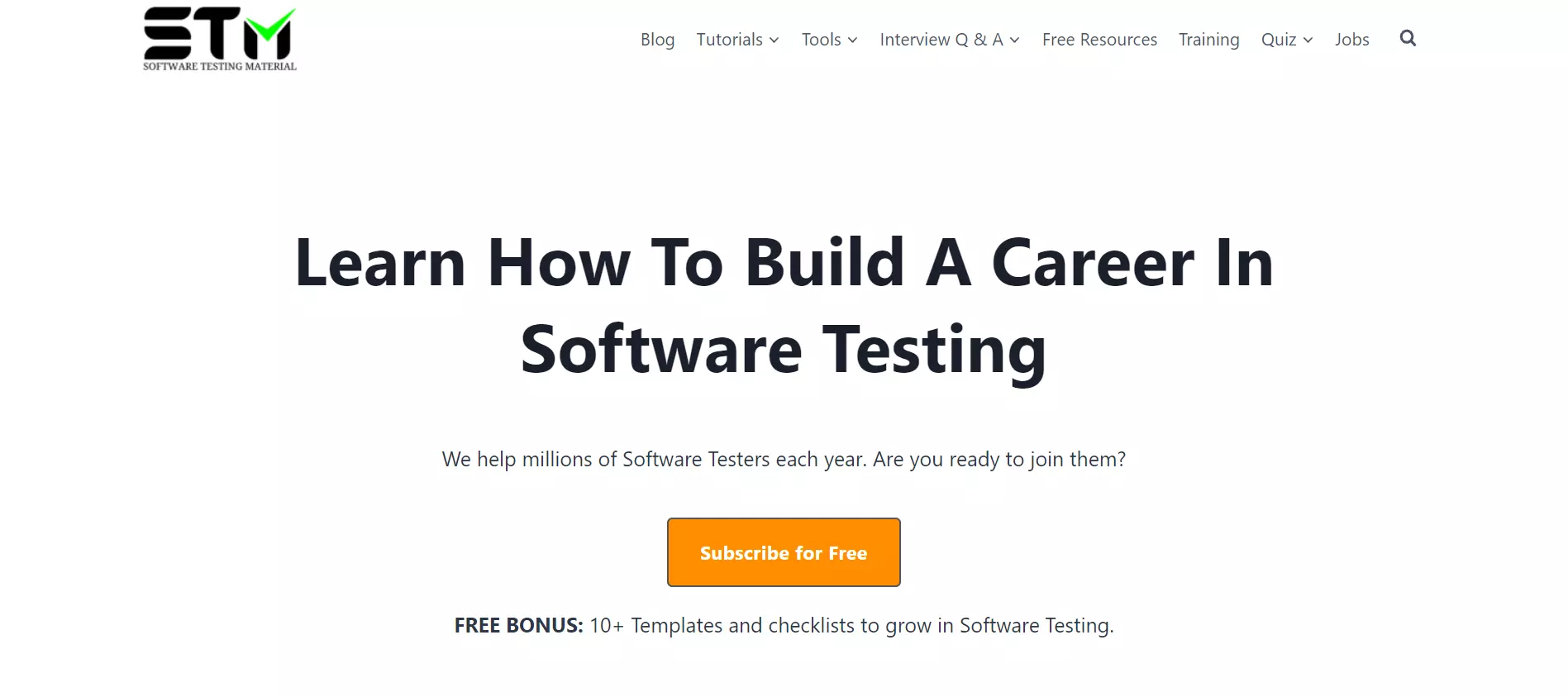
FAQ
What is the difference between Selenium Screenshot feature and the new Selenium full page screenshot feature?
Selenium screenshot feature captures the visible part of the web page. Whereas, Selenium full page screenshot feature captures the entire webpage.
Where the full page screenshot can be used?
The full page screenshot feature in Selenium 4 can be used in bug analysis and reporting.
Conclusion
Here we have seen the full-page screenshot feature released newly in Selenium 4.
getScreenshotAs() – This Selenium 4 method allows us to capture a screenshot of a particular WebElement.
getFullPageScreenshotAs() – This method of Selenium 4 allows us to take full page screenshot in Firefox.
Related posts:
Excellent. All articles are very meaningful and informative based on purely practical implementation. Heartiest thanks for providing your knowledge. Please keep it up.
Thanks for your kind words.