WebDriver driver = new FirefoxDriver() – Why we write in Selenium Scripts
In this post, we see why in Selenium scripts we write WebDriver driver = new FirefoxDriver(). Many students asked this question and I would like to share few points here.
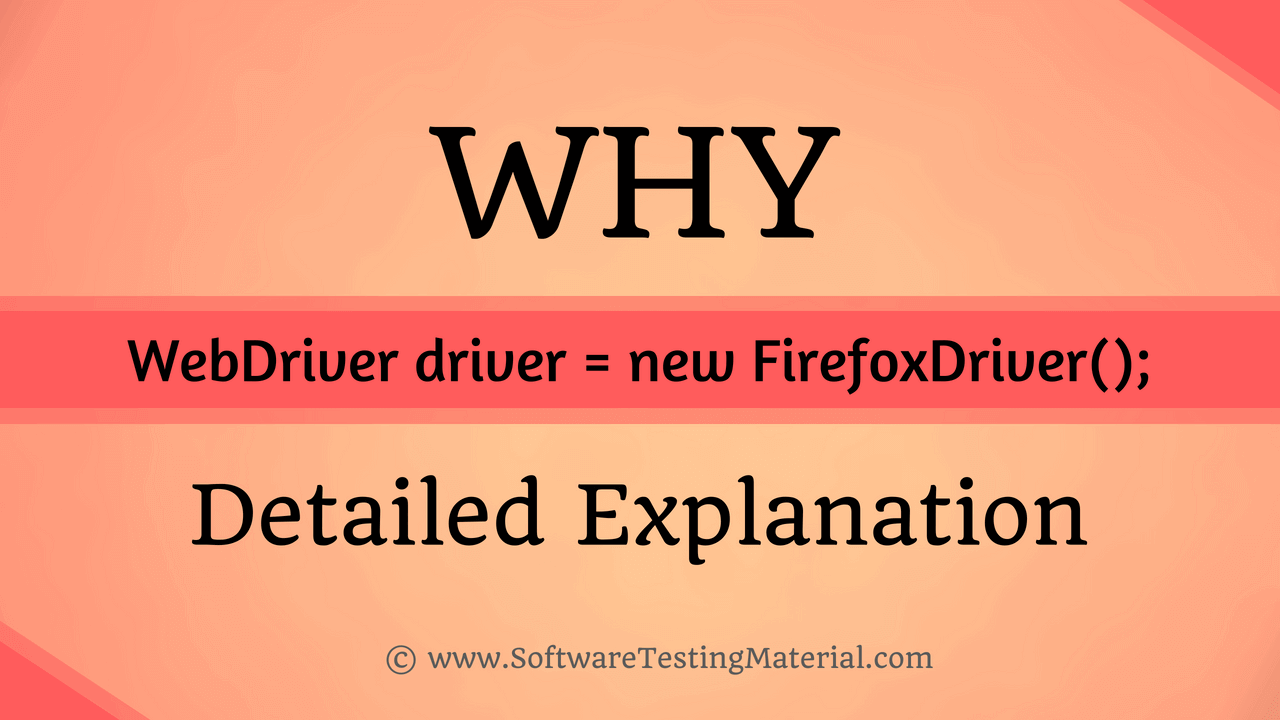
We are going to discuss following points in this post.
Before going into the details lets see the below image.
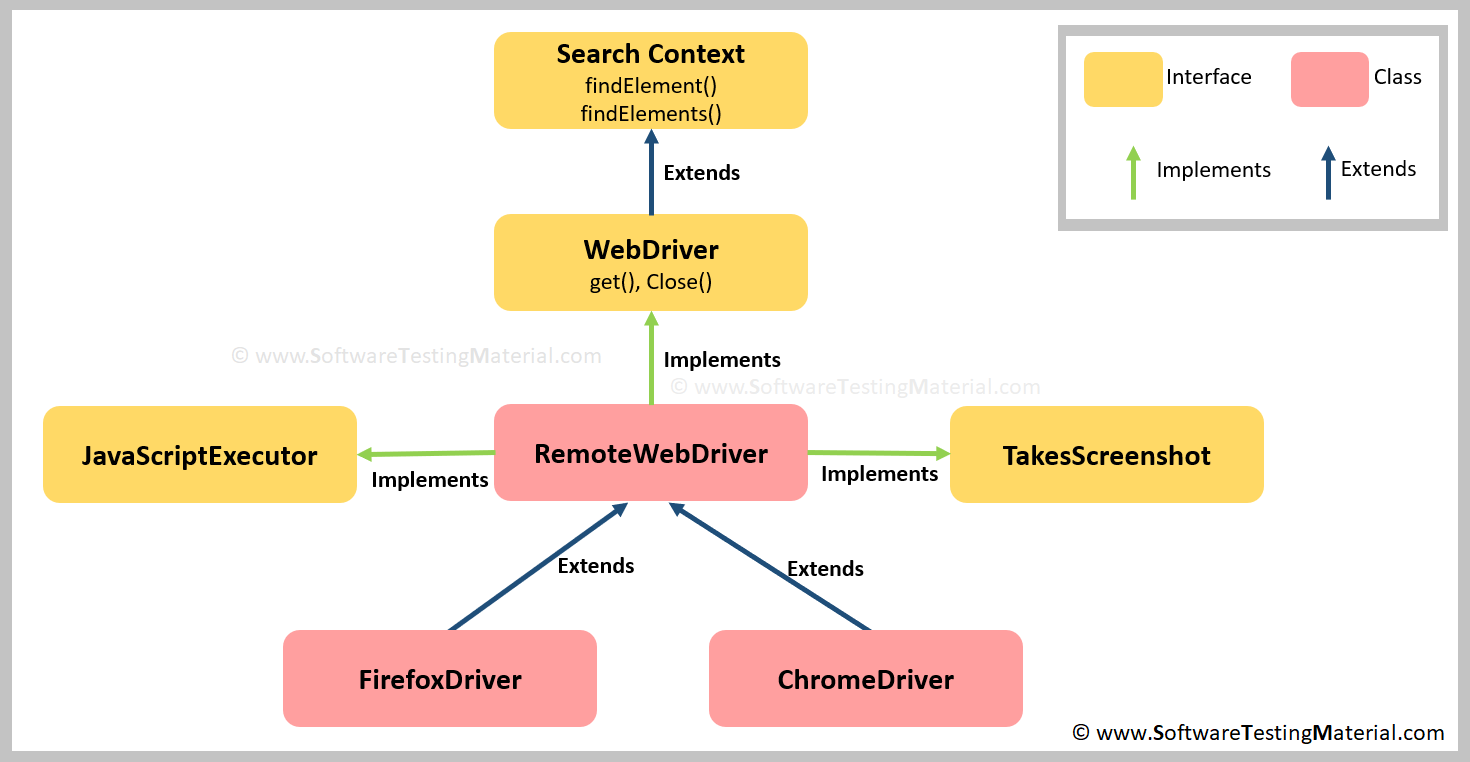
Following are the few points based on the above image.
- SearchContext is the super most interface in selenium, which is extended by another interface called WebDriver.
- All the abstract methods of SearchContext and WebDriver interfaces are implemented in RemoteWebDriver class.
- All the browser related classes such as FirefoxDriver, ChromeDriver etc., extends the RemoteWebdriver class.
WebDriver defines common methods which all browser classes (such as Firefox, Chrome etc.,) use. All these class methods are derived from WebDriver interface.
All the abstract methods of both the interfaces are implemented in RemoteWebDriver class which is extended by browser classes such as Firefox Driver, Chrome Driver etc.
Why not WebDriver driver = new WebDriver()
Let’s see why can’t we use the following statement.
WebDriver driver = new WebDriver();
We cannot write our code like this because we cannot create Object of an Interface. WebDriver is an interface.
But we can use any of the following statements in our script
FirefoxDriver driver = new FirefoxDriver();
or
WebDriver driver = new FirefoxDriver();
Let’s see both of them in detail.
Why we wont prefer FirefoxDriver driver = new FirefoxDriver()
FirefoxDriver driver = new FirefoxDriver();
The FirefoxDriver instance which gets created based on above statement will be only able to invoke and act on the methods implemented by FirefoxDriver and supported by Firefox Browser only. We know that FirefoxDriver is a class and it implements all the methods of WebDriver interface. Using this statement, we can run our scripts only on Firefox Browser.
To act with other browsers we have to specifically create individual objects as below:
ChromeDriver driver = new ChromeDriver();
InternetExplorerDriver driver = new InternetExplorerDriver();
We don’t just run our scripts only on single browser. We use multiple browsers for Cross Browser Compatibility. We need the flexibility to use other browsers like ChromeDriver() to run on Chrome Browser and InternetExplorerDriver() to run on IE Browser and so on.
So, once you initiate a Firefox browser using FirefoxDriver driver = new FirefoxDriver();Â same object cannot be used to initiate Chrome Browser (you have to rename it)
Why WebDriver driver = new FirefoxDriver()
ChromeDriver driver = new ChromeDriver();
To solve this we use “Webdriver driver = new FirefoxDriver();“
Lets see this now.
WebDriver driver = new FirefoxDriver();
We can create Object of a class FirefoxDriver by taking reference of an interface (WebDriver). In this case, we can call implemented methods of WebDriver interface.
As per the above statement, we are creating an instance of the WebDriver interface and casting it to FirefoxDriver Class. All other Browser Drivers like ChromeDriver, InternetExplorerDriver, PhantomJSDriver, SafariDriver etc implemented the WebDriver interface (actually the RemoteWebDriver class implements WebDriver Interface and the Browser Drivers extends RemoteWebDriver). Based on this statement, you can assign Firefox driver and run the script in Firefox browser (any browser depends on your choice).
We will see RemoteWebDriver in later section below.
If you define driver as a WebDriver, switching will be very easy. If we use this statement in our script then the WebDriver driver can implement any browser. Every browser driver class implements WebDriver interface and we can get all the methods. It helps you when you do testing on multiple browsers.
Example:
WebDriver driver = new FirefoxDriver();
driver.quit();
driver = new ChromeDriver();
WebDriver is an interface and all the methods which are declared in Webdriver interface are implemented by respective driver class. But if we do upcasting,we can run the scripts in any browser .i.e running the same automation scripts in different browsers to achieve Runtime Polymorphism.
WebDriver driver = new FirefoxDriver();
Here, WebDriver is an interface, driver is a reference variable, FirefoxDriver() is a Constructor, new is a keyword, and new FirefoxDriver() is an Object.
General information: Selenium WebDriver is an Interface which contains different methods (eg., get(), getTitle(), close() etc., ). All the third party Browser vendors implement these methods in addition to their browser specific methods. This would in-turn help the end-users to use the exposed APIs to write a common code and implement the functionalities across all the available Browsers without any change. Selenium developers don’t know how all these browsers work. So Selenium developers just declare methods whatever they required and leave the implementation part to the browser developers.
Difference between WebDriver and RemoteWebDriver
As per Java’s interface concept, Interface contains only Method’s signature and it doesn’t contain the Method’s definitions.
Check this post to know the difference between Method Declaration and Method Definition.
WebDriver is the Interface which contains all the Selenium Method signatures (Eg findElement(),switchTo(),get() etc.) where as definition for those methods are in RemoteWedDriver Class.
If we want to run our automation scripts on the local machine’s browser then we can use any class (such as Firefoxdriver, iedriver, chromedriver, htmlunitdriver) except RemoteWebDriver. WebDriver will start up a web browser on the computer where the code instantiates it.
If we want to run our automation scripts on the remote machine’s browser then we use RemoteWebDriver. RemoteWebDriver requires the Selenium Standalone Server to be running but to use other drivers Selenium Standalone Server is not required.
If you want to work with Grid then you have to stick with RemoteWebDriver. The only requirement is that for a RemoteWebDriver to work, you would always have to have it pointing to the URL of a Grid.
If you are using any of the drivers other than RemoteWebDriver then the communication will happen on the local machine’s browser.
Ex: Webdriver driver = new FirefoxDriver();
driver will access Firefox on the local machine, directly.
What is RemoteWebDriver and where we use it
If we use RemoteWebDriver then we have to mention where the Selenium Server is located and which web browser you want to use.
Ex: WebDriver driver = new RemoteWebDriver(new URL Desired capabilities.firefox());
We can use RemoteWebDriver the same way we would use WebDriver locally. The primary difference is that remote webdriver needs to be configured so that it can run your tests on a remote machine.
WebDriver is an interface that you should be using throughout your tests. RemoteWebDriver is a concrete implementation of that interface. In general, its always a good idea to code against interfaces wherever possible.
Hope you are clear. If you like this post, don’t forgot to share it.
You may also like this:
- Dynamic XPath – Complete Guide
- JavaScriptExecutor – Complete Guide
- How to get the advantage of BrowserStack in Selenium
- Learn API Testing in 10 mins
Popular Interview Questions
- Selenium Interview Questions
- Java Interview Questions
- Software Testing Interview Questions
- Agile Testing Interview Questions
- SQL Interview Questions
Firefox drive is not a constructor. It is a class
Go through the below link u should be able to get details.
http://seleniumhq.github.io/selenium/docs/api/java/index.html
Hi Ramani, Yes FirefoxDriver is a class. Even I mentioned that in my post. Also check why I mentioned FirefoxDriver is a constructor. Hope you didnt go through the complete post. Please read it again. If you still have any queries, please comment here.