What Are Selenium Relative Locators And How To Use Them
In this Selenium 4 series, we will dive into Selenium Relative locators.
What are Relative Locators in Selenium 4?
Relative Locators in Selenium formerly known as Friendly Locators.
In test automation, the automation tester our main task while writing test scripts for web applications is to locate web elements. We already know the existing Selenium locators (id, name, className, linkText, partialLinkText, tagName, cssSelector, xpath). You can learn about Selenium locators here. The point to be noted here is sometimes we may not find elements using these locators and to overcome that we use JavaScriptExecutor. You can learn about JavaScriptExecutor in Selenium here.
In Selenium 4.0, a new locator added to the locator’s list i.e., Friendly locators and later it is renamed as Relative locators.
It helps us to locate the web elements by its position by concerning other web elements such as above, below, toLeftOf, toRightOf, and near.
In simple words, relative locators allow us to locate the web elements based on their position with respect to other web elements.
There are five locators newly added in Selenium 4:
above()
It is to locate a web element just above the specified element
below()
It is to locate a web element just below the specified element
toLeftOf()
It is to locate a web element present on the left of a specified element
toRightOf()
It is to locate a web element present on the right of a specified element
near()
It is to locate a web element at approx. 50 pixels away from a specified element. The distance can be passed as an argument to an overloaded method.
Note: Method “withTagName()” is added which returns an instance of RelativeLocator. Above relative locators support this method “withTagName()”
Practical Example Selenium Relative Locators
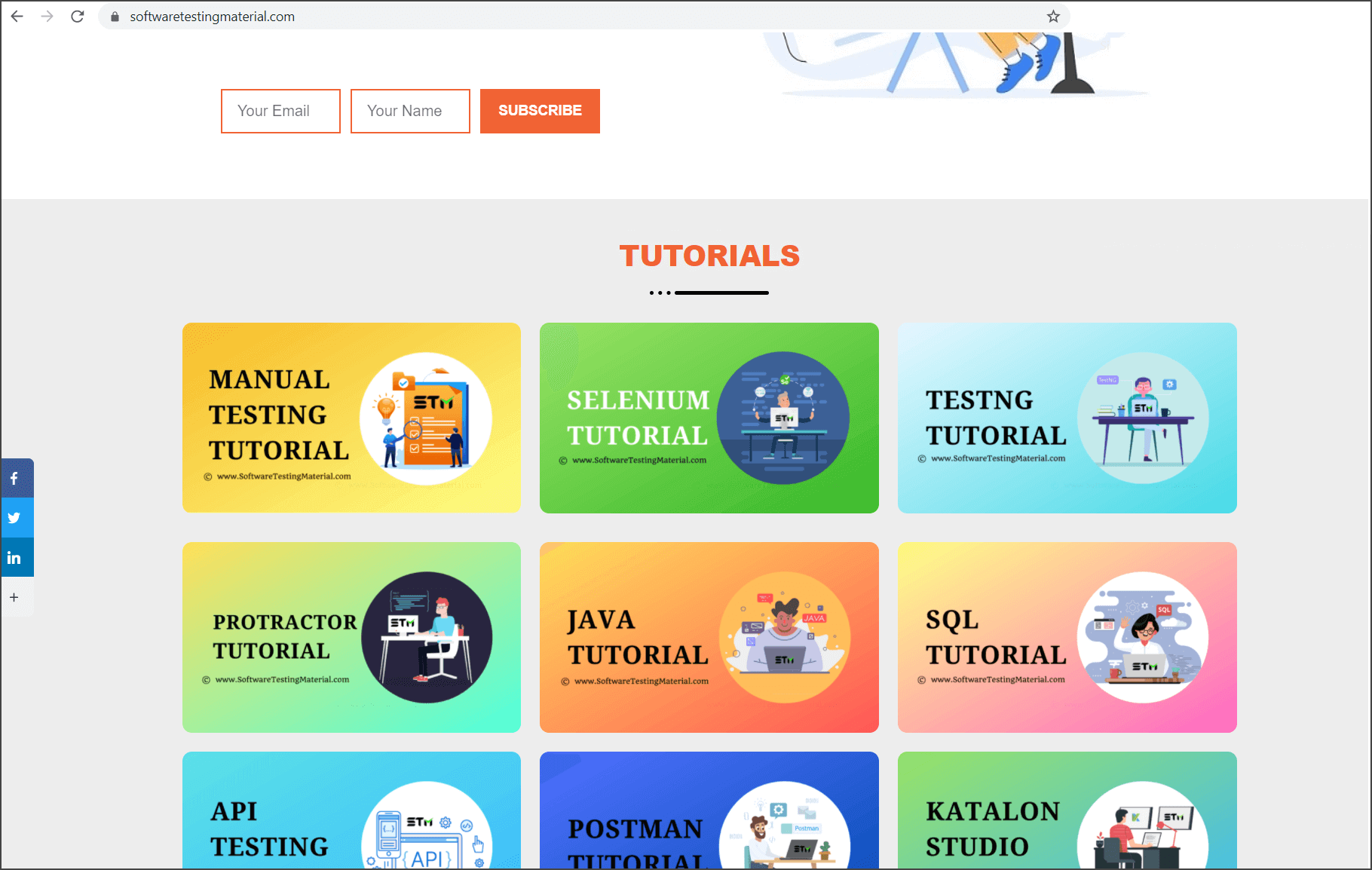
STEP 1: Import RelativeLocator ‘withTagName’
import static org.openqa.selenium.support.locators.RelativeLocator.withTagName;
STEP 2: Launch a web browser and navigate to https://www.softwaretestingmaterial.com/
STEP 3: Locate the web element ‘Your Name’ text field
STEP 4: Locate the web element ‘Your Email’ text field
STEP 5: Locate ‘Your Email’ text field which is to the left of ‘Your Name’ text field and enter the text
STEP 6: Locate ‘Your Name’ text field which is to the right of ‘Your Email’ text field and enter the text
STEP 7: Locate the web element ‘Selenium Tutorial’ and open it
STEP 8: Locate the web element ‘Java Tutorial’ and open it
STEP 9: Close the browser to end the program
package softwaretestingmaterial; import static org.openqa.selenium.support.locators.RelativeLocator.withTagName; import java.util.List; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.firefox.FirefoxDriver; public class RelativeLocatorsSample { public static void main(String[] args) { System.setProperty("webdriver.gecko.driver","C:\\Selenium\\drivers\\geckodriver-v0.24.0-win64\\geckodriver.exe"); WebDriver driver = new FirefoxDriver(); //Launch a web browser and navigate to https://www.softwaretestingmaterial.com/ driver.get("http://softwaretestingplace.blogspot.com/2015/10/sample-web-page-to-test.html"); //Locate the web element 'Your Name' text field WebElement yourNameLabel = driver.findElement(By.xpath("//input[@id='form-field-name']")); //Locate the web element 'Your Email' text field WebElement yourEmailLabel = driver.findElement(By.xpath("//input[@id='form-field-email'])); //Locate 'Your Email' text field which is to the left of 'Your Name' text field and enter the text WebElement txtEmailLabel = driver.findElement(withTagName("input").toLeftOf(yourNameLabel)); txtEmailLabel.sendKeys("email@email.com"); //Locate 'Your Name' text field which is to the right of 'Your Email' text field and enter the text WebElement txtNameLabel = driver.findElement(withTagName("input").toRightOf(yourEmailLabel)); txtNameLabel.sendKeys("YourName"); //Locate the web element 'Selenium Tutorial' and open it WebElement seleniumTutorial = driver.findElement(withTagName("img").below(subject)); seleniumTutorial.click(); //Locate the web element 'Java Tutorial' and open it WebElement javaTutorial = driver.findElement(withTagName("img").above(subject)); javaTutorial.click(); //Close the browser driver.close(); } }
Related Posts: