Python Multiline String
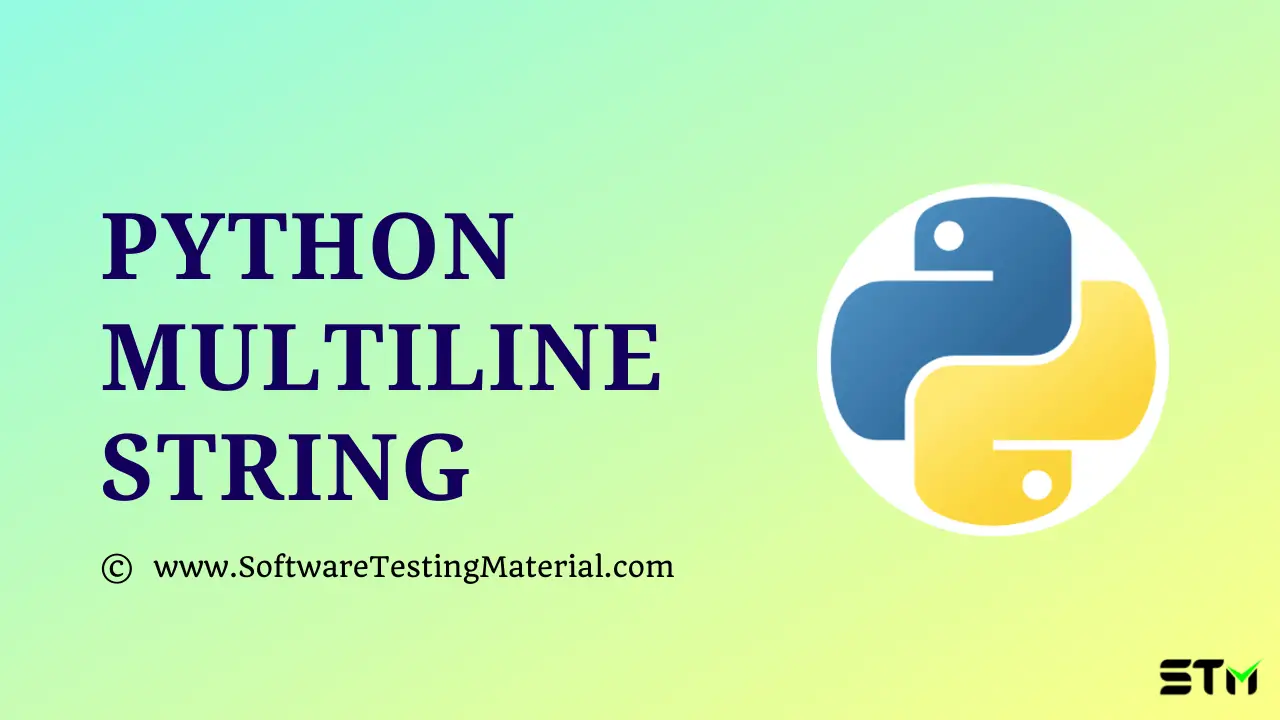
In this article, we will see various ways of creating “Python Multiline Strings”. It will help you in implementing this concept and get a tight grip over this.
Imagine you have a very long string that needs to be used in your program. Keeping all the text in a single line makes it difficult to read and looks clumsy. It gives better readability if you write it into multiple lines. Multiline String in python helps you to overcome this.
Also, learn Strings in Python here.
Multiline String in Python
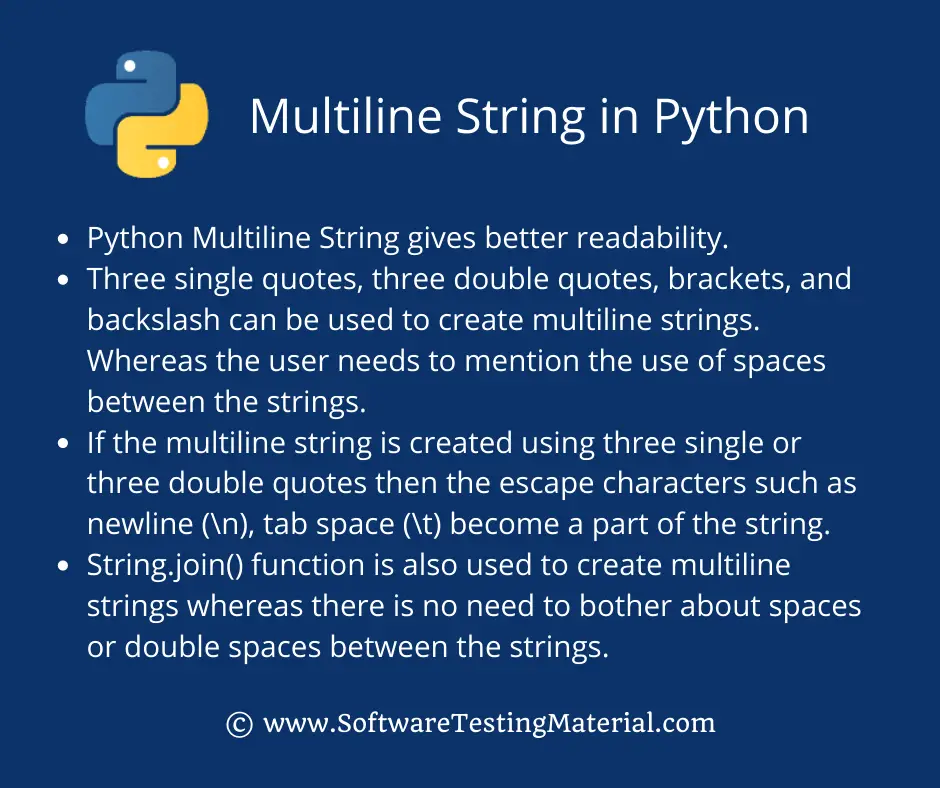
You can assign a multiline string to a variable in the following ways:
We have a long string as follows
x = 'I am learning Python.\nI love Software Testing Material.\nMultiline String in Python.'
1. By using three double quotes
Python multiline string begins and ends with either three single quotes or three double-quotes.
Open the file editor and write the following
Example:
x = """I am learning Python. I love Software Testing Material. Best site to learn Python.""" print(x)
Save the program and run it.
Output:
I am learning Python. I love Software Testing Material. Best site to learn Python.
2. By using three single quotes
Example:
x = '''I am learning Python. I love Software Testing Material. Easy way to learn Python''' print(x)
Output:
I am learning Python. I love Software Testing Material. Easy way to learn Python.
Incase the String doesn’t have newline characters, we have to implement it in other ways to write in multiple lines.
Note: Using three single quotes or three double quotes, the escape characters such as newline (\n), tab space (\t) become a part of the string.
3. Using Brackets
Using brackets we can split a string into multiple lines.
Example:
x = ("I am learning Python. " "I love Software Testing Material. " "Learn Python in a simple way.") print(x)
Output:
I am learning Python. I love Software Testing Material. Learn Python in a simple way.
4. Using Backslash
Using backslash we can split a string into multiple lines in Python. In Python, backslash works as a line continuation character. We use it to join text which is separate lines.
Example:
x = "I am learning Python." \ "I love Software Testing Material." \ "Cool Python Tips." print(x)
Output:
I am learning Python. I love Software Testing Material. Cool Python Tips.
5. Multiline String creation using join()
Using string join() function, we can split a string into multiple lines. The advantage of using join() function in python over brackets or backslash way is there is no need to worry about the spaces or double spaces.
Example:
x = ' '.join(("I am learning Python.", "I love Software Testing Material.", "Using Join Function in Python.")) print(x)
Output:
I am learning Python. I love Software Testing Material. Using Join Function in Python.
Summary
- Python Multiline String gives better readability.
- Three single quotes, three double quotes, brackets, and backslash can be used to create multiline strings. Whereas the user needs to mention the use of spaces between the strings.
- If the multiline string in python is created using three single or three double quotes then the escape characters such as newline (\n), tab space (\t) become a part of the string.
- String.join() function is also used to create multiline strings whereas there is no need to bother about spaces or double spaces between the strings.
Also Read: